javascript
javascript rest
SharePoint get by Rest List Role DefinitionBindings
On 28/10/2024
let currentSort = { column: null, direction: 'asc' }; // Store the current sort state
// Creates the style element
function createStyleElement(id, content) {
var style = document.createElement("style");
style.type = "text/css";
style.id = id;
style.innerHTML = content;
if (style.styleSheet) {
style.styleSheet.cssText = content;
} else {
let st = document.getElementById(id);
if (st == undefined) {
var head = document.head || document.getElementsByTagName("head")[i];
head.appendChild(style);
} else {
st.innerHTML = content;
}
}
return style;
}
// Function to filter the table based on dropdown selection
function filterTable(columnIndex, value) {
let table, tr, td, i, select, selectedValue, txtValue;
table = document.querySelector("table");
tr = table.getElementsByTagName("tbody")[0].getElementsByTagName("tr");
select = table.getElementsByTagName("select")[columnIndex];
//debugger;
selectedValue = value;
// Loop through all table rows and hide those that don't match the filter
for (i = 0; i < tr.length; i++) {
td = tr[i].getElementsByTagName("td")[columnIndex];
if (td) {
txtValue = td.textContent || td.innerText;
if (selectedValue === "" || txtValue === selectedValue) {
tr[i].style.display = "";
} else {
tr[i].style.display = "none";
}
}
}
}
function sortTable(columnIndex, direction) {
let table, rows, switching, i, x, y, shouldSwitch;
table = document.querySelector("table");
switching = true;
let tbody = table.querySelector("tbody");
// Set the current sort state
currentSort.column = columnIndex;
currentSort.direction = direction;
while (switching) {
switching = false;
rows = tbody.rows;
for (i = 0; i < rows.length - 1; i++) {
shouldSwitch = false;
x = rows[i].getElementsByTagName("td")[columnIndex];
y = rows[i + 1].getElementsByTagName("td")[columnIndex];
let isNumber = false;
if (!isNaN(x.innerHTML)) {
// Check if rows should switch based on ascending or descending order
if (direction === 'asc') {
if (parseFloat(x.innerHTML) > parseFloat(y.innerHTML)) {
shouldSwitch = true;
break;
}
} else if (direction === 'desc') {
if (parseFloat(x.innerHTML) < parseFloat(y.innerHTML)) {
shouldSwitch = true;
break;
}
}
}
else {
// Check if rows should switch based on ascending or descending order
if (direction === 'asc') {
if (x.innerHTML.toLowerCase() > y.innerHTML.toLowerCase()) {
shouldSwitch = true;
break;
}
} else if (direction === 'desc') {
if (x.innerHTML.toLowerCase() < y.innerHTML.toLowerCase()) {
shouldSwitch = true;
break;
}
}
}
}
if (shouldSwitch) {
rows[i].parentNode.insertBefore(rows[i + 1], rows[i]);
switching = true;
}
}
}
// Function to generate the table
function generateTableFromJson2(jsonArray, select, addHeaders = true) {
const style = `
table {
width: 100%;
border-collapse: collapse;
}
th, td {
padding: 8px 12px;
text-align: left;
border: 1px solid #ddd;
}
tbody tr{
max-height: 15px;
}
th {
background-color: #f4f4f4;
color: #000;
}
/* Scrollable table wrapper */
.table-wrapper {
max-height: 800px;
overflow-y: auto;
border: 1px solid #ddd;
}
/* Style for dropdowns in header */
select {
width: 100%;
padding: 4px;
margin-top: 5px;
}
/* Style for the sorting arrows */
.sort-arrows {
cursor: pointer;
margin-left: 5px;
}
`;
createStyleElement("fdiStyle", style);
// Create table element
let table = document.createElement('table');
// Create table header
let header = table.createTHead();
let headerRow = header.insertRow(0);
// Get keys (headers) from the first object in the JSON array
//let keys = Object.keys(jsonArray[0]);
let keys = select.split(",");
if (addHeaders) {
keys.forEach((key, index) => {
if (key !== "__metadata") {
let th = document.createElement('th');
th.innerHTML = key;
// Create a dropdown (select) for filtering
let select = document.createElement('select');
select.addEventListener('change', function () {
const selectedValue = select.value;
filterTable(index, selectedValue);
});
// Populate dropdown with unique values from the JSON data
let uniqueValues = [...new Set(jsonArray.map(item => item[key]))];
// Add a default "All" option for no filter
let optionAll = document.createElement('option');
optionAll.value = "";
optionAll.text = `All`;
select.appendChild(optionAll);
// Create an option for each unique value
if (typeof (uniqueValues[0]) === typeof (1)) {
const pp = uniqueValues.sort((a, b) => {
if (a < b) {
return -1;
}
if (a > b) {
return 1;
}
return 0;
});
pp.forEach(value => {
let option = document.createElement('option');
option.value = value;
option.text = value;
select.appendChild(option);
});
} else
uniqueValues.sort().forEach(value => {
let option = document.createElement('option');
option.value = value;
option.text = value;
select.appendChild(option);
});
// Sort arrows for sorting the columns
let upArrow = document.createElement('span');
upArrow.innerHTML = '⬆️';
upArrow.classList.add('sort-arrows');
upArrow.onclick = () => sortTable(index, 'asc');
let downArrow = document.createElement('span');
downArrow.innerHTML = '⬇️';
downArrow.classList.add('sort-arrows');
downArrow.onclick = () => sortTable(index, 'desc');
th.appendChild(select); // Append the dropdown to the header
th.appendChild(upArrow); // Append the dropdown to the header
th.appendChild(downArrow); // Append the dropdown to the header
headerRow.appendChild(th);
}
});
}
// Create table body and populate rows with data
let tbody = document.createElement('tbody');
jsonArray.forEach((item) => {
let row = tbody.insertRow();
keys = select.split(",");
keys.forEach((key) => {
let cell = row.insertCell();
if (key !== "__metadata") {
cell.setAttribute("nowrap", "nowrap");
if (key === "RoleDefinitionBindings") {
cell.appendChild(generateTableFromJson2(item.RoleDefinitionBindings.results, "Name,Id", false));
} else if (key.indexOf("/") > 0) {
cell.innerHTML = item[key.split("/")[0]][key.split("/")[1]]
} else
cell.innerHTML = item[key]; // Insert each value from the JSON into the table cell
}
});
});
// Append the body to the table
table.appendChild(tbody);
return table;
}
function removeSlasches(select, datas) {
const ret = [];
const fields = select.split(',');
for (let i = 0; i < datas.length; i++) {
const toAdd = {};
for (let j = 0; j < fields.length; j++) {
if (fields[j].indexOf('/') > 0) {
const splitted = fields[j].split('/');
toAdd[splitted.join('')] = datas[i][splitted[0]][splitted[1]];
} else
toAdd[fields[j]] = datas[i][fields[j]];
}
ret.push(toAdd);
}
console.log("removeSlasches", ret);
return ret;
}
// Function to get permissions for a SharePoint group
async function getSharePointPermissions(siteUrl, select) {
// REST API endpoint to get group permissions
const endpoint = `${siteUrl}`;
// Fetch options with headers for authentication and response format
const fetchOptions = {
method: 'GET',
headers: {
'Accept': 'application/json;odata=verbose'
}
};
console.log("endpoint", endpoint);
// Send the asynchronous GET request to the REST API endpoint
const response = await fetch(endpoint, fetchOptions);
// Check if the response is OK (status code 200)
if (!response.ok) {
throw new Error(`Error fetching permissions: ${response.statusText}`);
}
// Parse the JSON response to extract permission data
const data = await response.json();
// Extract role assignments (permissions)
const roleAssignments = data.d.results;
console.log('roleAssignments', roleAssignments);
console.log(JSON.stringify(roleAssignments));
const D1 = removeSlasches(select, roleAssignments)
const pattern2 = /\//g;
console.log("json111", JSON.stringify(D1));
const table = generateTableFromJson2(D1, select.replace(pattern2, ""));
// Append the table to the container
document.getElementById('tableContainer').appendChild(table);
}
// Usage example: Replace 'your-group-id' and 'your-site-url' with actual values
const select = "Member/Title,Member/Id,Member/LoginName,RoleDefinitionBindings";
let siteUrl = 'https://mySite.sharepoint.com/sites/Dev_wf';
siteUrl += `/_api/web/roleassignments/?$expand=RoleDefinitionBindings,Member&$select=${select}`; // Replace with the actual site URL
document.body.innerHTML = `<div id="tableContainer" class="table-wrapper"></div>`; await getSharePointPermissions(siteUrl, select);
Get User Permissions By Mail And Javascript
On 09/09/2024
Get User Permissions By Mail And Javascript
let siteUrl = "https://mayTenant.sharepoint.com/sites/test"
async function getUserPermissions(siteUrl, userMail) {
// REST API endpoint to get group permissions
const endpoint = `${siteUrl}/_api/web/siteusers?$filter=Email eq '${userMail}'&$select=Id`;
//const endpoint = `${siteUrl}/_api/web/siteusers?$filter=Email eq '${userMail}'&$select=LoginName`;
// Fetch options with headers for authentication and response format
const fetchOptions = {
method: 'GET',
headers: {
'Accept': 'application/json;odata=verbose'
}
};
console.log("endpoint", endpoint);
// Send the asynchronous GET request to the REST API endpoint
const response = await fetch(endpoint, fetchOptions);
// Check if the response is OK (status code 200)
if (!response.ok) {
throw new Error(`Error fetching permissions: ${response.statusText}`);
}
// Parse the JSON response to extract permission data
const data = await response.json();
console.log("data", data);
if (data.d.results.length === 0) {
console.log("user not found", userMail);
} else {
const userId = `${data.d.results[0].Id}`;
for (const user in data.d.results) {
console.log("user", userMail);
}
let ret = "";
const endpoint2 = `${siteUrl}/_api/web//RoleAssignments/GetByPrincipalId(${userId})/RoleDefinitionBindings`;
// Send the asynchronous GET request to the REST API endpoint
const response2 = await fetch(endpoint2, fetchOptions);
const data2 = await response2.json();
console.log("data2", data2);
for (let i = 0; i < data2.d.results.length; i++) {
ret += `User ${data2.d.results[0].Name} : ${data2.d.results[0].Description}\r\n`;
}
//by groups
const getGroupsEndPoint = `${siteUrl}/_api/web/GetUserById(${userId})/groups?$select=Id,Title`;
const response3 = await fetch(getGroupsEndPoint, fetchOptions);
const data3 = await response3.json();
console.log("data3", data3);
for (let i = 0; i < data3.d.results.length; i++) {
//get group permissions
const endpoint4 = `${siteUrl}/_api/web//RoleAssignments/GetByPrincipalId(${data3.d.results[i].Id})/RoleDefinitionBindings`;
const response4 = await fetch(endpoint4, fetchOptions);
const data4 = await response4.json();
console.log("data4", data4);
for (let j = 0; j < data4.d.results.length; j++) {
ret += `Group ${data3.d.results[i].Title} Id ${data3.d.results[i].Id} : ${data4.d.results[j].Name} : ${data4.d.results[j].Description}. ` + "\r\n";
}
}
return `${ret}.`;
}
return null;
}
await getUserPermissions(siteUrl, "test-ext@test.com");
Permissions to Check:
Here are some common permissions encoded in the High and Low values:
- ViewListItems: 0x00000001
- AddListItems: 0x00000002
- EditListItems: 0x00000004
- DeleteListItems: 0x00000008
- OpenItems: 0x00000010
- ViewVersions: 0x00000020
- CancelCheckout: 0x00000040
- ManagePermissions: 0x00010000
- ManageWeb: 0x00040000
SharePoint List Users And Their Groups By JS to CSV
On 06/09/2024
let siteUrl = '';
async function getUsersGroups(siteUrl) {
// REST API endpoint to get group permissions
const endpoint = `${siteUrl}`;
// Fetch options with headers for authentication and response format
const fetchOptions = {
method: 'GET',
headers: {
'Accept': 'application/json;odata=verbose'
}
};
console.log("endpoint", endpoint);
// Send the asynchronous GET request to the REST API endpoint
const response = await fetch(endpoint, fetchOptions);
// Check if the response is OK (status code 200)
if (!response.ok) {
throw new Error(`Error fetching permissions: ${response.statusText}`);
}
// Parse the JSON response to extract permission data
const data = await response.json();
console.log("data", data);
let csv = "Title;Email;LoginName;Id;IsSiteAdmin;UserOnly;GroupTitle;GroupId;Owner;Description;AllowMembersEditMembership;AllowRequestToJoinLeave;AutoAcceptRequestToJoinLeave;GroupId;Limited\r\n";
for (const user of data.d.results) {
//debugger;
if (user.Groups && user.Groups.results && user.Groups.results.length > 0) {
csv += `${user.Title};${user.Email};${user.LoginName};${user.Id};${user.IsSiteAdmin};true;;;;;;;;;NoLimited\r\n`;
for (const group of user.Groups.results) {
let limited = "not"
if (`${group.Title}`.indexOf("imited") > 0) {
limited = "Limited";
}
csv += `${user.Title};${user.Email};${user.LoginName};${user.Id};${user.IsSiteAdmin};group;${group.Title};${group.Id};${group.OwnerTitle};${group.Description};${group.AllowMembersEditMembership};${group.AllowRequestToJoinLeave};${group.AutoAcceptRequestToJoinLeave};${group.Id};${limited}\r\n`;
}
} else
csv += `${user.Title};${user.Email};${user.LoginName};${user.Id};${user.IsSiteAdmin};noGroup;;;;;;;;;noGroup\r\n`;
}
console.log("csv", csv);
}
let query = `/_api/web/siteusers?$select=IsSiteAdmin,Email,Id,LoginName,Title&$expand=Groups`;
await getUsersGroups(siteUrl + query);
On 05/09/2024
Display Sharepoint lists in an html table, and in a csv text content
// The content of the stylesheet
const styleSheetContent = `
.cadre-table-scroll {
display: inline-block;
height: 100em;
overflow-y: scroll;
overflow-x: auto;
white-space: nowrap;
}
.table-scroll thead th {
position: sticky;
top: 0;
}
.table-scroll tfoot td {
position: sticky;
bottom: 0;
}
.table-scroll tbody {
display: table;
width: 100%;
}
`;
// Creates the style element
function createStyleElement(id, content) {
var style = document.createElement("style");
style.type = "text/css";
style.id = id;
style.innerHTML = content;
if (style.styleSheet) {
style.styleSheet.cssText = content;
} else {
let st = document.getElementById(id);
if (st == undefined) {
var head = document.head || document.getElementsByTagName("head")[i];
head.appendChild(style);
} else {
st.innerHTML = content;
}
}
return style;
}
async function getSharePointLists(query) {
const endpoint = `${query}`;
// Fetch options with headers for authentication and response format
const fetchOptions = {
method: 'GET',
headers: {
'Accept': 'application/json;odata=verbose'
}
};
console.log("endpoint", endpoint);
// Send the asynchronous GET request to the REST API endpoint
const response = await fetch(endpoint, fetchOptions);
// Check if the response is OK (status code 200)
if (!response.ok) {
throw new Error(`Error fetching permissions: ${response.statusText}`);
}
// Parse the JSON response to extract permission data
const data = await response.json();
let csv = "Id;Title;RootFolderr;BaseTemplate;ContentTypesEnabled;Created;ItemCount;EnableMinorVersions;EnableModeration;EnableVersioning;LastItemModifiedDate;NoCrawl;Hidden\r\n";
// Extract lists
const lists = data.d.results;
console.log("lists", lists);
createStyleElement("fdiStyle", styleSheetContent);
let html = `<table class="cadre-table-scroll"><thead><tr>
<th>Id</th>
<th>Title</th>
<th>RootFolderr/ServerRelativeUrl</th>
<th>BaseTemplate</th>
<th>ContentTypesEnabled</th>
<th>Created</th>
<th>ItemCount</th>
<th>EnableMinorVersions</th>
<th>EnableModeration</th>
<th>EnableVersioning</th>
<th>LastItemModifiedDate</th>
<th>NoCrawl</th>
<th>Hidden</th></tr><thead><tbody>
`;
for (let i = 0; i < lists.length; i++) {
const responseList = await fetch(`${lists[i].__metadata.id}?$select=HasUniqueRoleAssignments`, fetchOptions);
const data = await responseList.json();
//console.log("responseList", data);
csv += `${lists[i].Id};${lists[i].Title};${lists[i].RootFolder.ServerRelativeUrl};${lists[i].BaseTemplate};${lists[i].ContentTypesEnabled};${lists[i].Created};${lists[i].ItemCount};${lists[i].EnableMinorVersions};${lists[i].EnableModeration};${lists[i].EnableVersioning};${lists[i].LastItemModifiedDate};${lists[i].NoCrawl};${lists[i].Hidden}`;
csv += `\r\n`;
if (lists[i].Title === "DO_NOT_DELETE_SPLIST_SITECOLLECTION_AGGREGATED_CONTENTTYPES")
continue;
lists[i].HasUniqueRoleAssignments = data.d.HasUniqueRoleAssignments;
html += `<tr>
<td>${lists[i].Id}</td>
<td>${lists[i].Title}</td>
<td>${lists[i].RootFolder.ServerRelativeUrl}</td>
<td>${lists[i].BaseTemplate}</td>
<td>${lists[i].ContentTypesEnabled}</td>
<td>${lists[i].Created}</td>
<td>${lists[i].ItemCount}</td>
<td>${lists[i].EnableMinorVersions}</td>
<td>${lists[i].EnableModeration}</td>
<td>${lists[i].EnableVersioning}</td>
<td>${lists[i].LastItemModifiedDate}</td>
<td>${lists[i].NoCrawl}</td>
<td>${lists[i].Hidden}</td>
</tr>`;
}
html += "</tbody></table>"
document.body.innerHTML = html;
console.log("datas", csv);
}
let req = "/_api/web/lists?$select=Id,Title,RootFolder/ServerRelativeUrl,ItemCount,BaseTemplate,ContentTypesEnabled,Created,EnableMinorVersions,EnableModeration,EnableVersioning,LastItemModifiedDate,NoCrawl,Hidden&$expand=RootFolder&$OrderBy=Hidden,Title";
let siteUrl = ";
getSharePointLists(siteUrl + req);
Get SharePoint Web Permissions
On 05/09/2024
Gets sharepoint roleassignements and display page, and createcsv text
// The content of the stylesheet
const styleSheetContent = '
.container {
display: grid;
row-gap: 10px;
/* 20px gap between rows */
column-gap: 10px;
/* 10px gap between columns */
grid-template-columns: 1fr 1fr 1fr 1fr;
padding-bottom: 5px;
}
.container2 {
display: grid;
row-gap: 10px;
/* 20px gap between rows */
column-gap: 10px;
/* 10px gap between columns */
grid-template-columns: 1fr 1fr 1fr;
padding-bottom: 5px;
}
.container .header {
text-align: center;
border: 1px solid black;
}
.container .data {
border: 1px solid black;
}
';
// Creates the style element
function createStyleElement(id, content) {
var style = document.createElement("style");
style.type = "text/css";
style.id = id;
style.innerHTML = content;
if (style.styleSheet) {
style.styleSheet.cssText = content;
} else {
let st = document.getElementById(id);
if(st == undefined){
var head = document.head || document.getElementsByTagName("head")[0];
head.appendChild(style);
} else {
st.innerHTML = content;
}
}
return style;
}
// Function to get permissions for a SharePoint group
async function getSharePointPermissions(siteUrl) {
// REST API endpoint to get group permissions
const endpoint = '${siteUrl}';
// Fetch options with headers for authentication and response format
const fetchOptions = {
method: 'GET',
headers: {
'Accept': 'application/json;odata=verbose'
}
};
console.log("endpoint", endpoint);
// Send the asynchronous GET request to the REST API endpoint
const response = await fetch(endpoint, fetchOptions);
// Check if the response is OK (status code 200)
if (!response.ok) {
throw new Error('Error fetching permissions: ${response.statusText}');
}
// Parse the JSON response to extract permission data
const data = await response.json();
// Extract role assignments (permissions)
const roleAssignments = data.d.results;
console.log('roleAssignments: ', data.d.results);
createStyleElement("fdiStyle", styleSheetContent);
let repportCSV = 'Title;LoginName;Id;Role_Name;Role_Description;Role;Id';
repportCSV += "\r\n";
document.body.innerHTML = '<div class="container">
<div class="header">Title</div>
<div class="header">LoginName</div>
<div class="header">Id</div>
<div class="header">Role
<div class="container2">
<div class="data">Name</div>
<div class="data">Description</div>
<div class="data">Id</div>
</div>
</div>
</div>';
// Report permissions
roleAssignments.forEach(roleAssignment => {
const member = roleAssignment.Member;
const roleBindings = roleAssignment.RoleDefinitionBindings.results;
repportCSV+= '${member.Title};${member.LoginName};${member.Id}';
let div = '
<div class="container">
<div class="data">${member.Title}</div>
<div class="data">${member.LoginName}</div>
<div class="data">${member.Id}</div>';
roleBindings.forEach(role => {
repportCSV+= ';${role.Name};${role.Description};${role.Id}';
repportCSV += "\r\n";
div += '
<div class="container2">
<div class="data">${role.Name}</div>
<div class="data">${role.Description}</div>
<div class="data">${role.Id}</div>
</div>
';
console.log(' - Role: ${role.Name}');
});
div += "";
document.body.innerHTML += div;
});
console.log("datas", repportCSV);
}
// Usage example: 'your-site-url' with actual values
let siteUrl = '';
siteUrl += '/_api/web/roleassignments/?$expand=RoleDefinitionBindings,Member&$select=Member/Title,Member/Id,Member/LoginName'; // Replace with the actual site URL
getSharePointPermissions(siteUrl);
On 05/09/2024
#### Introduction
Le développement de composants pour SharePoint Framework (SPFx) en utilisant React nécessite une attention particulière aux pratiques CSS pour garantir que vos interfaces sont responsives et adaptées à tous les types d'écrans, que ce soit sur mobile, tablette ou desktop.
#### 1. **Principes de Base pour des CSS Responsives**
1. **Unité Relative** :
- Utilisez des unités relatives comme `%`, `em`, `rem`, `vh`, et `vw` pour les marges, les paddings, et les dimensions. Cela permet aux éléments de s’adapter dynamiquement à la taille de l'écran.
2. **Media Queries** :
- Utilisez des media queries pour ajuster les styles en fonction de la taille de l'écran. Par exemple, pour les écrans de moins de 768px de largeur (taille de tablette), vous pouvez ajuster les layouts.
```css
@media (max-width: 768px) {
.container {
flex-direction: column;
}
}
```
3. **Flexbox** :
- Utilisez **Flexbox** pour créer des layouts flexibles qui peuvent s'adapter facilement aux différentes tailles d'écran. Flexbox est particulièrement utile pour les barres de navigation, les formulaires, et les mises en page de cartes.
```css
.form-container {
display: flex;
flex-wrap: wrap;
}
.form-group {
flex: 1 1 300px; /* Les groupes de formulaire s'ajustent avec un minimum de 300px */
margin: 10px;
}
```
4. **Grid Layout** :
- Pour des mises en page plus complexes, comme des tableaux de données ou des calendriers, utilisez **CSS Grid**. Cela permet de contrôler avec précision la disposition des éléments sur la page.
#### 2. **Exemple de Formulaire Responsive**
Voici un exemple de formulaire avec différents types d'entrées HTML, construit en React et avec une mise en page responsive.
##### **HTML/JSX pour le Formulaire**
```jsx
import * as React from 'react';
import './Form.css';
const ResponsiveForm = () => {
return (
<form className="form-container">
<div className="form-group">
<label htmlFor="name">Nom:</label>
<input type="text" id="name" name="name" placeholder="Votre nom" />
</div>
<div className="form-group">
<label htmlFor="email">Email:</label>
<input type="email" id="email" name="email" placeholder="Votre email" />
</div>
<div className="form-group">
<label htmlFor="date">Date:</label>
<input type="date" id="date" name="date" />
</div>
<div className="form-group">
<label htmlFor="color">Couleur:</label>
<input type="color" id="color" name="color" />
</div>
<div className="form-group">
<label>Sexe:</label>
<input type="radio" id="male" name="gender" value="male" />
<label htmlFor="male">Homme</label>
<input type="radio" id="female" name="gender" value="female" />
<label htmlFor="female">Femme</label>
</div>
<div className="form-group">
<label>Langues:</label>
<input type="checkbox" id="english" name="language" value="english" />
<label htmlFor="english">Anglais</label>
<input type="checkbox" id="french" name="language" value="french" />
<label htmlFor="french">Français</label>
</div>
<div className="form-group">
<label htmlFor="people-picker">Personne:</label>
<input type="text" id="people-picker" name="people-picker" placeholder="Sélectionner une personne" />
</div>
<div className="form-group">
<button type="submit">Envoyer</button>
</div>
</form>
);
};
export default ResponsiveForm;
```
##### **CSS pour le Formulaire**
```css
.form-container {
display: flex;
flex-wrap: wrap;
margin: 20px;
}
.form-group {
flex: 1 1 300px;
margin: 10px;
}
.form-group label {
display: block;
margin-bottom: 5px;
}
.form-group input[type="text"],
.form-group input[type="email"],
.form-group input[type="date"],
.form-group input[type="color"],
.form-group input[type="radio"],
.form-group input[type="checkbox"] {
width: 100%;
padding: 8px;
margin-bottom: 10px;
box-sizing: border-box;
}
button[type="submit"] {
background-color: #0078d4;
color: white;
padding: 10px 20px;
border: none;
cursor: pointer;
}
button[type="submit"]:hover {
background-color: #005a9e;
}
@media (max-width: 768px) {
.form-container {
flex-direction: column;
}
}
```
##### **Rendu Visuel**
L'utilisation de **Flexbox** ici permet aux groupes de formulaire de se réarranger dynamiquement en fonction de la largeur de l'écran. Sur des écrans larges, les groupes se placent côte à côte, tandis que sur les petits écrans (comme les mobiles), ils s'empilent verticalement.
#### 3. **Exemple de Grille Responsive avec les Jours de la Semaine**
Voici un exemple de grille responsive affichant les jours de la semaine.
##### **HTML/JSX pour la Grille**
```jsx
import * as React from 'react';
import './WeekGrid.css';
const WeekGrid = () => {
return (
<div className="week-grid">
<div className="day">Lundi</div>
<div className="day">Mardi</div>
<div className="day">Mercredi</div>
<div className="day">Jeudi</div>
<div className="day">Vendredi</div>
<div className="day">Samedi</div>
<div className="day">Dimanche</div>
</div>
);
};
export default WeekGrid;
```
##### **CSS pour la Grille**
```css
.week-grid {
display: grid;
grid-template-columns: repeat(7, 1fr);
gap: 10px;
margin: 20px;
}
.day {
background-color: #f4f4f4;
padding: 20px;
text-align: center;
font-weight: bold;
border: 1px solid #ddd;
}
@media (max-width: 768px) {
.week-grid {
grid-template-columns: repeat(2, 1fr);
}
}
@media (max-width: 480px) {
.week-grid {
grid-template-columns: 1fr;
}
}
```
##### **Rendu Visuel**
- **Grille par Défaut** : Les jours de la semaine sont affichés côte à côte sur des écrans larges, en 7 colonnes.
- **Tablette** : La grille passe à 2 colonnes, ce qui permet de voir deux jours par ligne.
- **Mobile** : La grille devient une seule colonne, chaque jour étant affiché dans une ligne distincte.
### Conclusion
Les bonnes pratiques pour des CSS responsives dans le développement SPFx avec React incluent l'utilisation de Flexbox et CSS Grid pour créer des mises en page adaptatives, l'utilisation judicieuse des media queries, et la préférence pour des unités relatives pour la taille des éléments. Ces techniques permettent de créer des interfaces utilisateurs qui sont à la fois élégantes et fonctionnelles sur tous types de dispositifs.
On 19/02/2024
PnP references
Get field in list
/_api/Web/Lists/getbytitle('Pages')/Fields?$select=InternalName,Id,Hidden,TypeAsString,Required&$filter=InternalName eq 'Title'
Get list content types
Get contentType fields
/_api/Web/Lists/getbytitle('Pages')/ContentTypes('0x010100C568DB52D9D0A14D9B2FDCC96666E9F2007948130EC3DB064584E219954237AF390028D78BF8D3054E38BEF0590B43C4BA0E00572E4F21E9A2274ABC0C3A6B4238D562')/fields?$filter=(Hidden eq false)and (Group ne '_Hidden')&$select= InternalName,Hidden,Required,Title,TypeAsString,Required,Id,Group
Query search
/_api/search/query?querytext='Path:https://mcn365.sharepoint.com/sites/a_IntranetPays_42/sitePages/*'&selectproperties='Title,Path'
/_api/search/query?querytext='owstaxIdJiveTags:GP0*+OR+owstaxIdJiveCategories:GP0*'&rowsperpage=0&rowlimit=5&selectproperties='Title%2cowstaxIdJiveCategories%2cowstaxIdJiveTags'&sortlist='Rank:descending%2cmodifiedby:ascending'&clienttype='ContentSearchRegular'">https://mcncs36sddcsdcsint.com/sites/a_IntranetPays_42/_api/search/query?querytext='owstaxIdJiveTags:GP0*+OR+owstaxIdJiveCategories:GP0*'&rowsperpage=0&rowlimit=5&selectproperties='Title%2cowstaxIdJiveCategories%2cowstaxIdJiveTags'&sortlist='Rank:descending%2cmodifiedby:ascending'&clienttype='ContentSearchRegular'
/_api/Web/Lists/getbytitle('Pages')/ContentTypes('0x010100C568DB52D9D0A1d37AF390028D78BF8D3054E38BEF0590B43C4BA0E00572E4F21E9A2274ABC0C3A6B4238D562')/fields?$filter=(Hidden eq false)and (Group ne '_Hidden')&$select= InternalName,Hidden,Required,Title,TypeAsString,Required,Id,Group
query user multi
/_api/web/lists/getbytitle('ResponsableRegions')/items(1)?$select=ResponsablesRegions/EMail,Id&$expand=ResponsablesRegions
get fields
/_api/web/lists/GetByTitle('Liste des chantiers')/fields?$filter=Hidden eq false&$orderby=Title&$select=Title,InternalName,TypeAsString,Hidden
On 05/04/2023
Create certification for azure app
# Create certificate
$mycert = New-SelfSignedCertificate -DnsName "myCertificate.org" -CertStoreLocation "cert:\CurrentUser\My" -NotAfter (Get-Date).AddYears(1) -KeySpec KeyExchange
$mypwd = ConvertTo-SecureString -String "myCertificatePsw" -Force -AsPlainText
# Export certificate to .pfx file
$mycert | Export-PfxCertificate -FilePath myCertificate.pfx -Password $mypwd
# Export certificate to .cer file
$mycert | Export-Certificate -FilePath myCertificate.cer
Connect to site
$url = "https://m365x6422vvvvd.sharepoint.com/";
$appId = "868d7a0c-a3dc-45af-b4a7-f72a70f61a60";
$thumbprint = "A17177BB0E8A465F6AD08B0CEAE2F369C46D6481";
$tenantId = "3533ab30-c2f0-48fd-b4c5-f5dc6ca77ec3"
Connect-PnPOnline -Url $url -Tenant $tenantId -Thumbprint $thumbprint -ClientId $appId
Export audit
premission required
Office 365 Management APIs (3) :: ActivityFeed.Read
possible filters
- FilePreviewed
- FileAccessed
- SignInEvent
- FileModifiedExtended
- FileUploaded
- PageViewed
- PagePrefetched
- FileCheckedIn
- FileModified
- FolderCreated
- ListUpdated
- ListViewed
$ele = Get-PnPUnifiedAuditLog -ContentType SharePoint -StartTime (Get-Date).AddDays(-2) -EndTime (Get-Date).AddDays(-1)
$ele = Get-PnPUnifiedAuditLog -ContentType SharePoint
$ele = Get-PnPUnifiedAuditLog -ContentType SharePoint | Where-Object {$_.Operation -eq "PageViewed"} | Select-Object CreationTime,Operation,Workload,UserId,ObjectId,SourceFileName,SiteUrl,SourceFileExtension,SourceRelativeUrl
$ele | Export-Csv -Path "Audit_3.csv" -Encodin:UTF8 -NoTypeInformation -Delimiter ";"
with sharepoint search request
kqlQuery = "ContentTypeId:0x0101009D1CB255DA76424F860D91F20E6C4118*";//news
kqlQuery = "ContentTypeId:0x0101* language=fr ViewsLastMonths3=0";//documents
kqlQuery = "ContentTypeId:0x0101* ViewsLifeTime=0";
var seletvvv = "Title,ViewsLifeTimeUniqueUsers,ViewsLifeTime,language,Created,Size,Path,LastModifiedTime,ViewsLastMonths3,ViewsLastMonths3Unique,LastAnalyticsUpdateTime";
&sortlist='Size:descending'
&sortlist='ViewsLifeTime:descending'
How to resolve the 5000 item limit
On 24/01/2022
var firstItems = listLocation.web.getList(listLocation.listUri).items.select("ID").top(1).orderBy("Id").get(); var lastItems = listLocation.web.getList(listLocation.listUri).items.select("ID").top(1).orderBy("Id", false).get(); if (firstItems.length === 1 && lastItems.length === 1) { const firstId: number = firstItems[0].ID; const lastId: number = lastItems[0].ID; let startId = firstId; let endId = firstId + 5000; do { let mySites: ISite[] = await listLocation.web.getList(listLocation.listUri).items .select("ID", "Title", "Url") .filter(`Id ge ${startId} and Id lt ${endId} and ProprietairesPeople/Id eq ${userId}`) .expand('ProprietairesPeople') .orderBy("Title") .top(5000) .getAll(); allMySites.push(...mySites); startId = startId + 5000; endId = endId + 5000; } while (endId < lastId); } return allMySites;
On 20/09/2021
Add User To Sharepoint Group With Rest
var fdi = fdi || {};
fdi.post = function(request, data, urlWeb, retFunction, expecedcode=200, odata="verbose"){
var url = _spPageContextInfo.webAbsoluteUrl + "/_api/contextinfo?&select=FormDigestValue";
var xhr = new XMLHttpRequest();
xhr.open('POST', url, true);
xhr.setRequestHeader("Accept", "application/json; odata=nometadata");
xhr.onload = function () {
if (xhr.status === 200) {
console.log(url + " : success");
var d = JSON.parse(xhr.responseText);
console.dir(d.FormDigestValue);
var viewXml = "" +
"" +
" " +
" " +
" ";
var req = { "query" :{"__metadata": { "type": "SP.CamlQuery" }, "ViewXml": viewXml}};
var xhrPOST = new XMLHttpRequest();
var reqUrl = _spPageContextInfo.webAbsoluteUrl + "/_api/web/sitegroups(5)/users";
//debugger;
xhrPOST.open('POST', reqUrl, true);
xhrPOST.setRequestHeader("Accept", "application/json; odata=" + odata);
xhrPOST.setRequestHeader("content-type", "application/json; odata=" + odata);
xhrPOST.setRequestHeader("X-RequestDigest", d.FormDigestValue);
console.log("onload");
xhrPOST.onload = function () {
console.log("onload ok");
console.dir(JSON.parse(xhrPOST.responseText));
}
var metadata = {
__metadata: {
'type': 'SP.User'
},
LoginName: 'i:0#.f|membership|fred.ddiet@test.com'
}; JSON.stringify(metadata)
xhrPOST.send(JSON.stringify(metadata));
console.log("sent");
}
else {
console.log("error");
console.log(xhr.status);
console.dir(xhr);
console.dir(xhr.responseText);
}
};
xhr.send();
};
fdi.post();
Get Sharepoint List Items Count
On 02/04/2021
Get Sharepoint list Items count
var xhr = new XMLHttpRequest();
console.clear();
var url = "https://mySiteUrl";
xhr.open('GET', url + "/_api/web/Lists/getbytitle('TheListName')?$select=ItemCount,Title");
xhr.setRequestHeader("Accept", "application/json; odata=verbose");
xhr.onload = function () {
if (xhr.status === 200) {
var kk = JSON.parse(xhr.responseText);
console.dir(kk.d.Title + " Item Count : " + kk.d.ItemCount);
}
else {
console.dir(xhr);
alert('Request failed. Returned status of ' + xhr.status);
}
};
xhr.send();
In your browser you must be your your site url, push key F12 paste above code in console, en push enter
fr
On 06/01/2021
Use Sharepoint REST api to do a caml query
fdi.post = function(request, data, urlWeb, retFunction, expecedcode=200, odata="verbose"){ var url = _spPageContextInfo.webAbsoluteUrl + "/_api/contextinfo?&select=FormDigestValue"; var xhr = new XMLHttpRequest(); xhr.open('POST', url, true); xhr.setRequestHeader("Accept", "application/json; odata=nometadata"); xhr.onload = function (data) { if (xhr.status === 200) { console.log(url + " : success"); var d = JSON.parse(xhr.responseText); console.dir(d.FormDigestValue); var viewXml = "
<View><Query>
" + " <Where></Where>
" + " </Query>
" + " </View>
" + "
"; var req = { "query" :{"__metadata": { "type": "SP.CamlQuery" }, "ViewXml": viewXml}}; var xhrPOST = new XMLHttpRequest(); var reqUrl = _spPageContextInfo.webAbsoluteUrl + "/_api/web/lists/GetByTitle('Catalogue Produits')/GetItems"; debugger; xhrPOST.open('POST', reqUrl, true); xhrPOST.setRequestHeader("Accept", "application/json; odata=" + odata); xhrPOST.setRequestHeader("content-type", "application/json; odata=" + odata); xhrPOST.setRequestHeader("X-RequestDigest", d.FormDigestValue); console.log("onload"); xhrPOST.onload = function () { console.log("onload ok"); console.dir(JSON.parse(xhrPOST.responseText)); } xhrPOST.send(JSON.stringify(req)); console.log("sent"); } else { console.log("error"); console.log(xhr.status); console.dir(xhr); console.dir(xhr.responseText); } }; xhr.send(); };
Sharepoint Modern Update listItem
On 09/12/2020
Update list in a modern site, getting formDigestValue
console.clear();
var dataToManage = {};
function getItemTypeForListName(listTitle, returnfct){
var xhr = new XMLHttpRequest();
var url = _spPageContextInfo.webAbsoluteUrl;
if (url === "undefined") {
console.log("_spPageContextInfo.webAbsoluteUrl undefined");
url = "http://siteUrl";
}
xhr.open('GET', url + "/_api/web/lists/getbytitle('" + listTitle + "')/?$select=ListItemEntityTypeFullName");
xhr.setRequestHeader("Accept", "application/json; odata=verbose");
xhr.onload = function () {
if (xhr.status === 200) {
var kk = JSON.parse(xhr.responseText);
console.dir(kk.d.ListItemEntityTypeFullName);
var returnValue = {};
returnValue.ListItemEntityTypeFullName = kk.d.ListItemEntityTypeFullName;
if(returnfct != null)
returnfct(returnValue);
}
else {
console.dir(xhr);
alert('Request failed. Returned status of ' + xhr.status);
}
};
xhr.send();
}
//get FormDigestValue to do POST requests
function GetDisgestValue(returnFct){
var url = _spPageContextInfo.webAbsoluteUrl + "/_api/contextinfo";
var xhr = new XMLHttpRequest();
xhr.open('POST', url, true);
xhr.setRequestHeader("Accept", "application/json; odata=verbose");
xhr.setRequestHeader("content-type", "application/json; odata=verbose");
xhr.onload = function (data) {
if (xhr.status === 200) {
console.log("success");
var d = JSON.parse(xhr.responseText);
console.dir(d.d.GetContextWebInformation.FormDigestValue);
dataToManage.FormDigestValue = d.d.GetContextWebInformation.FormDigestValue;
returnFct(d.d.GetContextWebInformation.FormDigestValue);
}
else {
console.log("error");
console.log(xhr.status);
console.dir(xhr);
console.dir(xhr.responseText);
}
};
xhr.send();
}
function UpdateListItem(){
var url = _spPageContextInfo.webAbsoluteUrl + "/_api/web/lists/getbytitle" +
"('" + dataToManage.listName + "')/items("+dataToManage.itemId+")";
var xhr = new XMLHttpRequest();
xhr.open('POST', url, true);
xhr.setRequestHeader("Accept", "application/json; odata=verbose");
xhr.setRequestHeader("X-RequestDigest", dataToManage.FormDigestValue );
xhr.setRequestHeader("content-type", "application/json; odata=verbose");
xhr.setRequestHeader("X-HTTP-Method", "MERGE");
xhr.setRequestHeader("If-Match", "*");
xhr.onload = function () {
if (xhr.status === 204) {
console.log("success");
}
else {
console.log("error");
console.log(xhr.status);
console.dir(xhr);
console.dir(xhr.responseText);
}
};
var str = JSON.stringify(dataToManage.item);
xhr.send(str);
}
function withDigestValue(formDigestValue){
console.log("formDigestValue : " + formDigestValue);
}
function getListDatas(data){
console.log(data.ListItemEntityTypeFullName);
dataToManage.listName = "aTest";//list title to update
dataToManage.itemId = 1;//id of the item to update
dataToManage.item = {
"__metadata": { "type": data.ListItemEntityTypeFullName },
"Title": "new title"//new fields values
};
GetDisgestValue(UpdateListItem);
}
getItemTypeForListName("atest", getListDatas);
Invalid Client Query Exception
On 07/07/2020
Microsoft.SharePoint.Client.InvalidClientQueryException A type named 'SP.Data' could not be resolved by the model. When a model is available, each type name must resolve to a valid type
get the correct ListItemEntityTypeFullName
var xhr = new XMLHttpRequest();
var url = _spPageContextInfo.webAbsoluteUrl;
if (url === "undefined") {
console.log("_spPageContextInfo.webAbsoluteUrl undefined");
url = "http://siteUrl";
}
//xhr.open('GET', url +"/_api/web/Lists?$select=Id,Title,Hidden,ItemCount");
xhr.open('GET', url + "/_api/lists/getbytitle('Campaigns')?$select=ListItemEntityTypeFullName");
xhr.setRequestHeader("Accept", "application/json; odata=verbose");
xhr.onload = function () {
if (xhr.status === 200) {
var kk = JSON.parse(xhr.responseText);
console.dir(kk);
}
else {
console.dir(xhr);
alert('Request failed. Returned status of ' + xhr.status);
}
};
xhr.send();
Sharepoint Update Item With REST
On 07/07/2020
Sharepoint update an item with REST no jquery
console.clear();
function UpdateListItem(listName){
var listItemId=176;
var itemType = "SP.Data.SurveysListItem";//GetItemTypeForListName(listName);
//debugger;
var item = {
"__metadata": { "type": itemType },
"LaunchReportingFlag": "0"
};
/*
"Reporting": ""
*/
var url = _spPageContextInfo.webAbsoluteUrl + "/_api/web/lists/getbytitle('" + listName + "')/items("+listItemId+")";
var requestdigest = document.getElementById("__REQUESTDIGEST");
var xhr = new XMLHttpRequest();
xhr.open('POST', url, true);
xhr.setRequestHeader("Accept", "application/json; odata=verbose");
xhr.setRequestHeader("X-RequestDigest", requestdigest.value);
xhr.setRequestHeader("content-type", "application/json; odata=verbose");
xhr.setRequestHeader("X-HTTP-Method", "MERGE");
xhr.setRequestHeader("If-Match", "*");
xhr.onload = function () {
if (xhr.status === 204) {
console.log("success");
}
else {
console.log("error");
console.log(xhr.status);
console.dir(xhr);
console.dir(xhr.responseText);
}
};
xhr.send(JSON.stringify(item));
}
UpdateListItem("Campaigns");
getItemTypeForListName
function getItemTypeForListName(listTitle){
var xhr = new XMLHttpRequest();
var url = _spPageContextInfo.webAbsoluteUrl;
if (url === "undefined") {
console.log("_spPageContextInfo.webAbsoluteUrl undefined");
url = "http://siteUrl";
}
//xhr.open('GET', url +"/_api/web/Lists?$select=Id,Title,Hidden,ItemCount");
xhr.open('GET', url + "/_api/web/lists/getbytitle('" + listTitle + "')/?$select=ListItemEntityTypeFullName");
xhr.setRequestHeader("Accept", "application/json; odata=verbose");
xhr.onload = function () {
if (xhr.status === 200) {
var kk = JSON.parse(xhr.responseText);
console.dir(kk.d.ListItemEntityTypeFullName);
}
else {
console.dir(xhr);
alert('Request failed. Returned status of ' + xhr.status);
}
};
xhr.send();
}
On 28/06/2020
use rest api to search items in sharepoint
var fdi = {};
fdi.search = function(url, query, target, selectProperties){
var req = url + "/_api/search/query?querytext='" + query + "'&selectproperties='"+ selectProperties +"'&sortlist='LastModifiedTime:descending'&rowlimit=500";
//&rowsperpage=0&rowlimit=5
var xhr = new XMLHttpRequest();
fdi.fdilog(req);
xhr.open('GET', req);
xhr.setRequestHeader("Accept", "application/json; odata=verbose");
xhr.onload = function () {
if (xhr.status === 200) {
var kk = JSON.parse(xhr.responseText);
fdi.lastQuery = req;
fdi.lastResult = kk;
fdi.fdilog(kk.d);
//debugger;
fdi.fdilog(kk.d.query.PrimaryQueryResult.RelevantResults.Table.Rows);//,TypeAsString,Title,Hidden,Required,Group
fdi.fdilog("RowCount : " + kk.d.query.PrimaryQueryResult.RelevantResults.RowCount);
fdi.fdilog("TotalRows : " + kk.d.query.PrimaryQueryResult.RelevantResults.TotalRows);
var rows = kk.d.query.PrimaryQueryResult.RelevantResults.Table.Rows.results;
var datas = [];
var columns = selectProperties.split(",");
for(var i = 0 ; i < rows.length ; i++){
var tar = {};
tar.rowNum = i;
for(var j = 0 ; j < columns.length ; j++){
var found = false;
for(var k = 0 ; k < rows[i].Cells.results.length ; k++){
if(rows[i].Cells.results[k].Key == columns[j]){
found = true;
tar[columns[j]] = rows[i].Cells.results[k].Value;
}
if(!found)
tar[columns[j]] = "";
}
}
datas.push(tar);
//rows[i].Cells.results[1].Key
}
fdi.fdilog(datas);
fdi.createTable("rowNum," + selectProperties, datas, target, "#EEEEEE");
}
else {
fdi.fdilog(req);
fdi.fdilog(xhr);
alert('Request failed. Returned status of ' + xhr.status);
}
};
xhr.send();//send request
}
use as below
var reqvvv = "ContentTypeId:0x010056313CD55A8D274EB8FAF2CA0B228BCA00AAEACC42FC8BD24797DEF621447F7D00*";
var seletvvv = "Title,Path,RefinableString20,RefinableString23,RefinableString24,RefinableString25,RefinableDecimal02,RefinableDecimal03,RefinableDecimal04,LastModifiedTime,ListItemID";
fdi.search("https://aSite/sites/Dev", reqvvv, "fdiOutPut", seletvvv);
Display in a table
fdi.createTable = function( columns, datas, target, backGroundColor){
if(fdi.isNullOrEmpty(target))
return;
// var targetDiv = document.getElementsByClassName("SPCanvas");
// targetDiv[0];
var targetDiv = document.getElementById(target);
targetDiv.innerHTML = "";
var tbl = document.createElement('table');
var att = document.createAttribute("class");
//var thead = document.createElement('thead');
var tr1 = document.createElement('tr');
var columnArray = columns.split(",");
var att = null;
for(var i = 0 ; i < columnArray.length ; i++){
var th = document.createElement('th');
fdi.fdidebuglog(columnArray[i]);
th.innerText = columnArray[i];
att = document.createAttribute("style");
att.value = "border:1px solid #000;width:230px";
th.setAttributeNode(att);
tr1.appendChild(th);
}
tbl.appendChild(tr1);
for(var j = 0 ; j < datas.length ; j++){
var tr2 = document.createElement('tr');
//background-color: #EEEEEE
if(j % 2 == 0 && typeof(backGroundColor) !== "undefined"){
att = document.createAttribute("style");
att.value = "background-color:" + backGroundColor + ";";
tr2.setAttributeNode(att);
}
for(var i = 0 ; i < columnArray.length ; i++){
var td = document.createElement('td');
td.innerText = datas[j][columnArray[i]];
att = document.createAttribute("style");
att.value = "border:1px solid #000;width:230px";
td.setAttributeNode(att);
tr2.appendChild(td);
}
tbl.appendChild(tr2);
}
targetDiv.appendChild(tbl);
}
On 15/05/2020
Sharepoint Query Search By UTC Date
On 09/12/2019
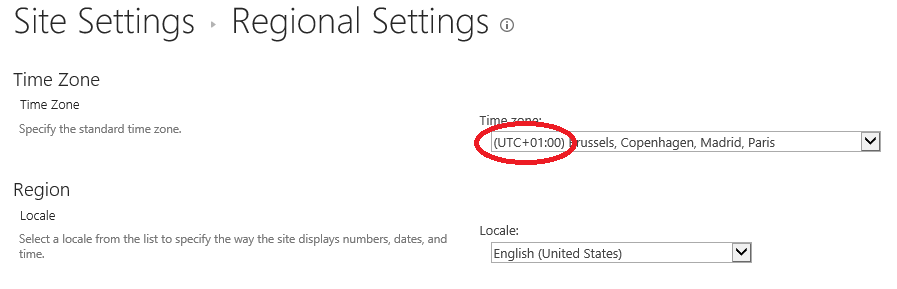
var aDate = new Date('2019-12-12);
var offset = new Date().getTimezoneOffset();
console.log("offset : " + offset);//in my case, offset is -60
console.dir(aDate);
aDate.setMinutes(aDate.getMinutes() + offset);
console.dir(aDate);
/_api/search/query='MnpDate:aDate.toISOString()';
// aDate : 2019-12-11T23:00:00.0000000Z
On 27/11/2019
Here is my page to develop my REST queries
if my query returns an object, it will display all properties of my obj, as in navigation console (console.dir(obj);)
if my query returns an array it will display all properties in input : "show properties'
complete code to add in a script editor webpart :see script
queries samples :
/_api/web/lists(guid'0E12917B-80F9-4765-8612-22A9AB467123')/items?$select=AssignedTo,Title&$orderby=DueDate desc&$filter=RelaunchDate eq null and Status eq 'In Progress'
/_api/web/getlist('/sites/csc-dev/Lists/myLMist')/fields?$select=Group,InternalName,Title,TypeAsString,Hidden
/_api/web/getlist('/sites/csc-dev/Lists/vdfdfvf')/fields?$select=Group,InternalName,Title,TypeAsString,Hidden&$filter=Hidden eq false and TypeAsString ne 'Computed' and startswith(InternalName, '_') eq false&$orderby=TypeAsString
/_api/web/getlist('/sites/csc-dev/Lists/myLMist')/fields?$select=Group,InternalName,Title,TypeAsString,Hidden
/_api/web/lists(guid'0E12917B-80F9-4765-8612-22A9AB46784E')/items?$select=AssignedTo/EMail,Created,Author/EMail,Body,DueDate,,ID,IsGroup,Modified,Editor/EMail,StartDate,Status,stepName,TaskGroup/EMail,TaskIdentifier,TaskProcessed,TaskProcessedBy/Title,TaskProcessedBy/Id,TaskProcessedBy/EMail,Title,WFFormFieldStepType,WFInstance&$orderby=DueDate desc&$filter=RelaunchDate eq null and Status eq 'In Progress'&$expand=Author,Editor,AssignedTo,TaskProcessedBy,TaskGroup
USER
{
Title: string;
EMail: string;
Id: number;
}
Sharepoint Query Search With REST
On 25/11/2019
On 30/10/2019
On 21/09/2019
Query sharepoint list with REST
use this end point to query your list http://test/sites/test/_api/Web/Lists/getbytitle('vvv')/items?$select=&$filter= Title eq 'entite du ga 1'
Example : http://test01/sites/test01/_api/Web/Lists/getbytitle('Aliste')/items?$select=Title,ID,Author/Title&$expand=Author&$filter= Title eq 'entite du toto 1'
to order by : &$orderby= Employee asc
to order by : &$orderby= Employee desc
to limit number of items returned : $top 5
to use paging : $skip 5
new paging mode add in your first query &$skiptoken=Paged=TRUE
in your response, you will receive next page query in odata.nextLink as below
/_api/Web/Lists/getbytitle(%27Initiatives%27)/items?%24skiptoken=Paged%3dTRUE%26p_ID%3d266&%24select=Title%2cID&%24orderby=ID+desc&p_ID=268&%24Top=3
expand lookup fields : $expand=city&$select=city/Id
viewfields : $select= Title, ID
filter : $filter=Title eq 'mon noeud' and ID ne 1
Numeric comparisons
- Lt
- Le
- Gt
- Ge
- Eq
- Ne
String comparisons
- startsWith
- substringof
- Eq
- Ne
Date and time functions
- day()
- month()
- year()
- hour()
- minute()
- second()
Full script to add in an Script Editor webpart
SPField Set ShowInDisplayForm With Rest
On 23/11/2018
Sharepoint ajax Without Jquery
On 16/11/2018
On 03/11/2018
Sharepoint Hide List With REST
On 10/10/2018
REST get Lists From Current Web
On 08/10/2018
On 29/09/2018